Trigonometric Fourier series representation#
In Section 6.9 of the book, we introduces the trigonometric Fourier series.
SymPy has a native function to compute trigonometric Fourier series. However, it uses a slightly different notation.
SymPy analyses the given signal in a finite period as opposed to the whole real axis (from minus infinity to plus infinity) as we did in the book.
In SymPy, the Fourier trigonometric series of \(x(t)\) over the interval \((l,u)\) is defined as
where the coefficients are as follows:
If you do not explicitly specify, the default range for \((l,u)\) is \((-\pi, \pi)\).
Let us find out the trigonometric Fourier series coefficients of \(x(t)=\cos(t)\).
import sympy as sym
t = sym.symbols('t', real=True)
x = sym.cos(t)
s = sym.fourier_series(x)
print("a_0: ", s.a0)
print("a_n (for n>=1): ", s.an)
a_0: 0
a_n (for n>=1): {1: 1}
The format {1:1}
means that \(a_1=1\) and all unspecified \(a_k\) are equal to
\(0\). All \(b_n\) are zeros:
print("b_n (for n>=1): ", s.bn)
b_n (for n>=1): {}
Let us use another signal:
x = 2 + sym.cos(t) + sym.sin(2*t)
s = sym.fourier_series(x)
print("a_0: ", s.a0)
print("a_n (for n>=1): ", s.an)
print("b_n (for n>=1): ", s.bn)
a_0: 2
a_n (for n>=1): {1: 1}
b_n (for n>=1): {2: 1}
Below we compute the trigonometric Fourier series of the following rectangular pulse signal:
with fundamental period \(2\).
x = sym.Heaviside(t+1/2) - sym.Heaviside(t-1/2)
sym.plot(x, (t, -1, 1))
s = sym.fourier_series(x, (t, -1, 1))
from IPython.display import display
display("a_0:", s.a0)
display("a_n (for n>=1):", s.an)
display("b_n (for n>=1):", s.bn)
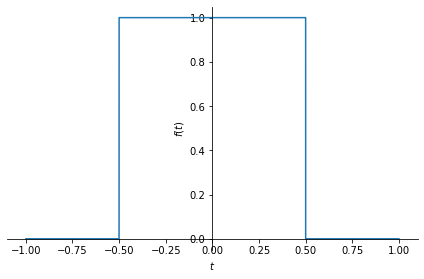
'a_0:'
'a_n (for n>=1):'
'b_n (for n>=1):'
Exercise: Write a script to convert trigonometric Fourier series coefficients to spectral coefficients (Hint: Section 6.9).
Related content:
Explore Fourier series representation for continuous time periodic signals.
An example on the duality of convolution and multiplication.